This tutorial introduces the new functionalities that were added to Entity Framework 4.1. It shows the differences between the approaches and acts as an introduction to these new features.
Database First & Model First
The Database First approach is interesting when the database already exists. You use Visual Studio and the Entity Framework Designer to generate the C# and VB.NET classes which reflect the existing database model.
You may then change relations and structures using the Designer (or the XML mapping files) to further optimize the model. The priority is the database - the code and the model are only secondary.
The Model First approach is important when you begin from scratch. You start with the entity model and use the Entity Framework Designer to design the relations and the entities.
Then you generate the C# and VB.NET classes and also the database from within Visual Studio. The priority is the model - the code and the database are only secondary.
Code First
When your priority is the code and you want to begin from scratch without any existing schemas or XML mapping files using source code, then the approach is called Code First.
You simply create your domain classes and Entity Framework 4.1 will easily allow to use them with the database and the future model.
You use a context and are able to execute Linq2Entities queries in no time ! You may even take advantage of the change tracking features !
Entity Framework handles all of the background work and manages the interaction with the database. All the classes that are used during runtime are auto-generated.
In this case the configuration is not read from XML files but instead read from the configuration of the DbContext object in memory.
Code First allows:
- Developing without the need to use the Designer or XML mapping files
- Defining the objects of the model based on a POCO (Plain Old CLR Objects) approach
Explanation:
- Design and implement a company domain with Managers, Collaborators and Departments.
- Create your classes using a standard object oriented approach and using inheritance when appropriate.
- Use the POCO approach for your classes where possible.
- Note that for being able to use the auto-mapping feature for the primary keys you need to have named your class properties like this : [Classname]Id.
- Entity Framework 4.1 allows connecting those POCO classes with the database very easily by using the ObjectContext class (and in particular the DbContext class).
- The last step consists of defining the connection to the database within the web.config or app.config files. Note that EF will search for the connection string that has the same name as the class that was inherited from the DbContext class. You may however define a different name ("CompanyContext" in our example).
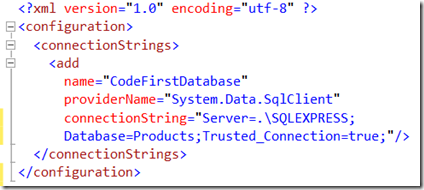

5 comments:
I am sorry there is a little error: As one of the blog visitors pointed out, the connection string name should be CompanyDatabase in the web.Config file.
Hi,
How can we avoid loosing data from tables when we make changes in models?
If we change in model classes then it's dropping DB and creating new DB.
Please help!
Thanks,
Anup M
Hello Anup,
Unfortunately there is no solution to this problem in EF 4.1, the DB will always get regenerated if the model structure changes.
You have to manually backup your data and restore it into the DB after regeneration if you want to avoid loosing your data.
This may change in future versions of EF but for now this is the only solution.
Also, you should not fill the DB with productive data until you are sure concerning the final structure.
Regards,
Jason De Oliveira
Hi,
I'm trying to use entity framework with an adapter structure, my goal will be to have a single .edmx structure able to manage several connections.
I need to have those adapters:
- file based database (SqlServerCE 3.5)
- MySql (with its custom provider from Oracle)
- Oracle (as MySql)
-SqlServer
I have a DAO class that receives a bean (dependency injection object) with data connection from a winform, than due to a specific info in that bean, the DAO will load the correct adapter, through a Database factory class.
My Database factory will load a real adaptor class (e.g. for mysql A_Mysql.cs that implement my abstract adapter class).
In particular, I would like to understand hop I can modify in the adaptor the connection method:
public override Entities createConnection(DbConnection dbBean)
{
string conn =
@"metadata=res://*/Toolkit.Database.External.ADO.ADODatabase.csdl" +
@"|res://*/Toolkit.Database.External.ADO.ADODatabase.ssdl" +
@"|res://*/Toolkit.Database.External.ADO.ADODatabase.msl;" +
@"provider=MySql.Data.MySqlClient;" +
"provider connection string=\"Persist Security Info=True;server=" + dbBean.Server + ";" +
"Port=" + dbBean.Port + ";" +
"User Id=" + dbBean.Username + ";"+
"Password=" + dbBean.Password + ";" +
"database=" + dbBean.Schema + "\"";
Entities entities = new Entities(conn);
return entities;
}
to use the same .edmx, in my DAO…
I was pretty sure that this was the right way, unfortunately this system is always returning me errors from SqlCE (I have generated the first .emdx from SqlServerCE, but it does not contain any informations about that database and my App.config file has NOT stored database informations)…
Can you help me? Please write me back for further information, if needed.
Thank you.
Hello Gian,
I will try to look into that as soon as possible and try to help you with this request.
Greetings,
Jason
Post a Comment