My last article introduced the new asynchronous features in C# 5.0. I took an easy development example that calculates factorials for showing you how you may implement it in a synchronous and asynchronous C# 4.0 environment - a time consuming and not so easy task for the common developer.
In this article I am going to explain how you could do it when using the latest C# 5.0 features that were released with the current CTP. You will see the code necessary to make your synchronous code asynchronous and how simple and comprehensible it is done when using the new async / await language features!
Factorials Asynchronous Code in C# 5.0
For being able to develop the C# 5.0 version of the code you need to have the CTP October Release installed and to reference the “AsyncCtpLibrary.dll”. Then you are ready to test the new features.
The main function gets a new section that calls the asynchronous C# 5.0 code. All three cases can now be executed from the main function for being able to compare the results.
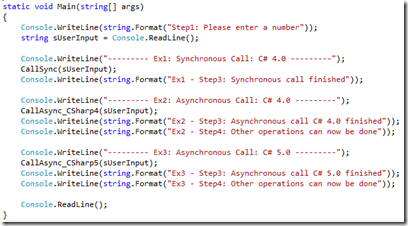
Now we need to implement the calling function that will start the asynchronous processing and wait for the asynchronous result. There are already changes in the function prototype since you need to add the async keyword before the function return type to mark the function to be asynchronous.
Furthermore you can see that I use the new await keyword in front of the function call which is done inside the function body. This will assure that the code inside of this function below this line is only executed when the asynchronous processing is finished. A calling function must have at least one and can have multiple await keywords.
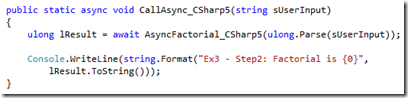
The main differences exist in the implementation of the function that will execute the factorials calculation code. You already see that the keyword async is used before the return type (similar to the function above).
But if you look carefully you can see that the Task<T> class which was introduced in C# 4.0 for Parallel Programming is used as return type. Knowing that all asynchronous functions have to either return Task or Task<T>, this indicates that the new asynchronous features work hand in hand with the TPL.
Within the function body we create an execute a new Task<T> and define the code that should run asynchronously, which is exactly the same that was used in all examples before.
Please also note the await keyword after the return statement that indicates that the return will only be done when all asynchronous operations within the function are completed.
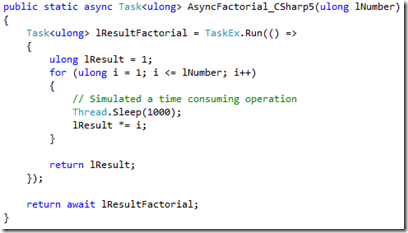
When executing the application the part that handles the asynchronous call makes that the factorial calculation is done in the background and the result is displayed when it is finished (similar to the example I presented in my last blog post).
Each step is marked in the logical order they are executed :
- Step1: Enter User Data
- Step2: Result of the calculation
- Step3: Exiting the function call
- Step4: Do some other stuff
When starting the application and entering a valid value you see that there is no more delay getting the output for Step3 and Step4 (as you have already seen for the C# 4.0 example). The application is not blocked by the processing which is done in Step2. When the asynchronous processing in Step2 is finished the result is written to the Command Line. That is why the execution order is different from the logical order.
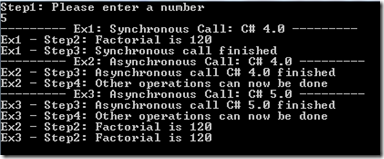
So the behavior is exactly the same but the code is much leaner, more elegant, easier to maintain and to understand. The async / await keywords greatly help to facilitate the migration of you synchronous code to its asynchronous version. No more hassle with synchronization contexts, delegates, events or wait handles. No more spaghetti code that has CallBacks allover the place where you may get very confused during design and even during runtime.
A developer may take this code and easily see what happens in which order. He may maintain or extends this kind of code very quickly. So in the end it leads to a higher productivity of developers who may concentrate once more on generating applications with high business value.
Conclusion
This article described the new features in C# 5.0 which will help to develop asynchronous code in a much quicker and cleaner way than it was possible before. Please take care that those functionalities are currently in CTP state so their final implementation may differ slightly from what I have shown you above. I will continue posting about those very interesting features and will give you updates if there are any changes in the final version of C# 5.0 which may still take some time to be released.
You can download the source code from the source code section of my Blog:

[Visual C# 5.0] How-To: Asynchronous Programming (2/2)